Harnessing the Power of Language Models for Text Classification
-
Andrew Butson
- 28 Jan, 2024
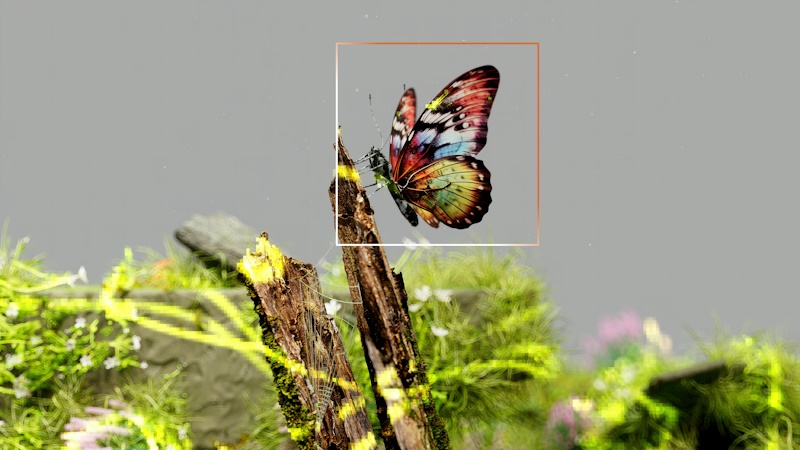
In today’s digital age, the explosion of textual data is both a blessing and a challenge. From social media posts and customer reviews to news articles and support tickets, organizations are inundated with text-based information. Making sense of this vast sea of data requires sophisticated tools, and that’s where natural language processing (NLP) and, more specifically, text classification come into play.
Text classification is the process of assigning predefined categories or labels to textual data. It’s the backbone of applications like spam detection, sentiment analysis, topic categorization, and intent detection. With the advent of advanced language models, our ability to classify and interpret text has grown exponentially.
In this article, we’ll explore how modern language models can be utilized for text classification tasks. We’ll delve into both representation models and generative models, illustrating their applications through engaging examples. Whether you’re a data scientist, an NLP enthusiast, or someone keen on leveraging AI for text analytics, this exploration promises valuable insights.
The Art of Text Classification
At its core, text classification involves teaching a model to recognize patterns in text and assign appropriate labels. Traditionally, this was achieved using methods like bag-of-words or TF-IDF representations combined with algorithms like Naive Bayes or Support Vector Machines. While effective to an extent, these approaches often struggled with understanding context and nuances in language.
Enter language models. These models, trained on vast amounts of textual data, have a deeper understanding of syntax, semantics, and even subtle linguistic cues. They can capture the intricacies of language in a way that traditional models cannot, making them ideal for complex classification tasks.
Exploring Language Models for Classification
There are two primary ways to leverage language models for text classification:
- Using Representation Models
- Using Generative Models
Let’s investigate each approach in detail.
1. Text Classification with Representation Models
Representation models, such as BERT (Bidirectional Encoder Representations from Transformers), RoBERTa, and DistilBERT, are designed to generate contextualized embeddings of text. These embeddings are numerical vectors that capture the meaning of words and sentences in context.
A. Task-Specific Models
Task-specific models are pretrained language models fine-tuned on specific classification tasks. For instance, a BERT model fine-tuned on sentiment analysis data can directly classify text as positive or negative.
Example: Sentiment Analysis of Movie Reviews
Imagine we want to classify movie reviews as positive or negative. We’ll use a pretrained model fine-tuned for sentiment analysis.
Steps:
-
Loading the Pretrained Model
from transformers import pipeline model_name = "nlptown/bert-base-multilingual-uncased-sentiment" sentiment_pipeline = pipeline("sentiment-analysis", model=model_name)
-
Classifying Reviews
reviews = [ "An absolute masterpiece. The storytelling was brilliant!", "I wasted two hours of my life watching this." ] results = sentiment_pipeline(reviews) for review, result in zip(reviews, results): print(f"Review: {review}\nSentiment: {result['label']}\n")
Output:
Review: An absolute masterpiece. The storytelling was brilliant!
Sentiment: 5 stars
Review: I wasted two hours of my life watching this.
Sentiment: 1 star
The model effectively classifies the reviews without additional training. This demonstrates the power of task-specific pretrained models in handling classification tasks out-of-the-box.
B. Using Embedding Models with Classifiers
Sometimes, a task-specific model for your particular classification problem may not be readily available. In such cases, you can use embedding models to generate representations of your text data and then train a classifier on top of these embeddings.
Example: Classifying News Articles by Topic
Suppose we’re tasked with categorizing news articles into topics like “Politics,” “Sports,” “Technology,” and “Entertainment.” We don’t have a pretrained model fine-tuned for this exact task, so we’ll use embeddings.
Steps:
-
Generating Embeddings
We’ll use the
SentenceTransformer
library to convert articles into embeddings.from sentence_transformers import SentenceTransformer model = SentenceTransformer('all-MiniLM-L6-v2') # Assume 'articles' is a list of article texts and 'labels' is a list of their corresponding topics embeddings = model.encode(articles, show_progress_bar=True)
-
Training a Classifier
We’ll train a logistic regression classifier using these embeddings.
from sklearn.linear_model import LogisticRegression from sklearn.model_selection import train_test_split X_train, X_test, y_train, y_test = train_test_split(embeddings, labels, test_size=0.2, random_state=42) classifier = LogisticRegression(max_iter=1000) classifier.fit(X_train, y_train)
-
Evaluating the Model
from sklearn.metrics import classification_report y_pred = classifier.predict(X_test) print(classification_report(y_test, y_pred))
By generating embeddings, we’ve transformed textual data into a format suitable for traditional machine learning classifiers. This approach is flexible and can be applied to various classification tasks.
2. Text Classification with Generative Models
Generative models, such as GPT-3 and T5, are designed to generate coherent and contextually relevant text. With clever prompt engineering, we can turn these models into powerful classifiers.
A. Using T5 Models
The Text-to-Text Transfer Transformer (T5) treats every NLP task as a text generation problem. It can be instructed to perform classification by framing the task appropriately.
Example: Classifying Support Tickets by Urgency
Consider a customer support system where incoming tickets need to be classified as “Low,” “Medium,” or “High” urgency.
Steps:
-
Creating Prompts
We’ll create a prompt that instructs the model to classify the ticket.
from transformers import T5ForConditionalGeneration, T5Tokenizer tokenizer = T5Tokenizer.from_pretrained('t5-small') model = T5ForConditionalGeneration.from_pretrained('t5-small') ticket = "Our website is down, and we cannot process any orders!" prompt = f"Classify the urgency of the following support ticket:\n\n{ticket}\n\nUrgency:"
-
Generating the Classification
inputs = tokenizer.encode(prompt, return_tensors='pt') outputs = model.generate(inputs, max_length=5) urgency = tokenizer.decode(outputs[0], skip_special_tokens=True) print(f"Predicted Urgency: {urgency}")
Output:
Predicted Urgency: High
By articulating the task as a prompt, the T5 model can perform classification without explicit fine-tuning on the dataset.
B. Leveraging GPT-3 for Zero-Shot Classification
GPT-3, with its extensive training data and parameters, is adept at understanding and generating human-like text. It can perform classification tasks even without prior exposure to specific datasets.
Example: Sentiment Analysis with GPT-3
Steps:
-
Crafting the Prompt
prompt = """ Determine the sentiment of the following review as Positive or Negative: "I absolutely love the new features in this update!" Sentiment: """
-
Generating the Response
Using OpenAI’s API (assuming you have access and an API key):
import openai openai.api_key = 'YOUR_API_KEY' response = openai.Completion.create( engine="text-davinci-003", prompt=prompt, max_tokens=1, temperature=0 ) sentiment = response.choices[0].text.strip() print(f"Sentiment: {sentiment}")
Output:
Sentiment: Positive
GPT-3 accurately classifies the sentiment based on the prompt. Adjusting the prompt allows us to handle a variety of classification tasks.
Comparing the Approaches
Each method has its advantages and considerations:
-
Task-Specific Models
- Pros: High accuracy when aligned with the task; straightforward to use.
- Cons: Limited to tasks they were fine-tuned on; may require fine-tuning for new tasks.
-
Embedding Models with Classifiers
- Pros: Flexible; can be applied to any text classification task; leverages traditional ML techniques.
- Cons: Performance depends on the quality of embeddings and the classifier.
-
Generative Models
- Pros: Capable of zero-shot and few-shot learning; highly versatile.
- Cons: May require careful prompt engineering; access to models like GPT-3 may involve costs.
Practical Considerations
When choosing an approach for text classification, consider the following:
- Data Availability: Do you have labeled data for fine-tuning or training a classifier?
- Task Specificity: Is your task general or domain-specific?
- Resource Constraints: Do you have the computational resources for fine-tuning large models?
- Infrastructure: Are you able to use external APIs, or do you need a solution that runs locally?
Example Scenario: Email Spam Detection
Let’s say an email service provider wants to implement spam detection. They can choose:
- Task-Specific Model: Fine-tune a model on a labeled dataset of spam and non-spam emails.
- Embedding Model: Generate embeddings of emails and train a classifier.
- Generative Model: Use a model like GPT-3 to assess emails based on prompts, useful if labeled data is scarce.
Future Directions
The field of NLP is rapidly evolving, with new models and techniques emerging regularly. Here are some areas to explore:
- Fine-Tuning Models: Experiment with fine-tuning language models on your specific datasets to improve performance.
- Prompt Engineering: Develop expertise in crafting prompts to elicit better responses from generative models.
- Hybrid Models: Combine the strengths of different models, such as using embeddings from one model with the generative capabilities of another.
Text classification is a foundational task in NLP with wide-ranging applications. The emergence of advanced language models has revolutionized how we approach this challenge. By harnessing the power of representation models and generative models, we can build sophisticated classifiers that understand language in deep and nuanced ways.
Whether deploying a pretrained task-specific model, generating embeddings for a traditional classifier, or leveraging the generative prowess of models like GPT-3, the tools at our disposal are more powerful than ever. The key is to understand the strengths and limitations of each approach and choose the one that best aligns with your specific needs and resources.